Seeq Formula Language
Overview
Seeq Workbench features a powerful calculation engine with an expressive language, called Formula, for manipulating signals (time series) and other data. Formula provides an efficient means to derive new, more valuable data sets, from original sources. Formula is designed to make it easy to filter, cleanse, aggregate, and otherwise improve large and disparate data sets to prepare for visualization and analysis.
This article provides an overview of the basic syntax of Formula. For a more detailed view of the functions available, as well as examples on how to use them, see the formula reference that is embedded in Workbench.
Basic Syntax
Seeq Formula is designed to make transformations of data – particularly signal data – simple and intuitive. The user is encouraged, through the use of variables, to make many intermediary transformations as opposed to compressing multiple transformations into a single unwieldy expression. The following example illustrates how two signals can be combined to create a third signal that represents the summation of the two for any moment in time.
If you have signal variables assigned as $a and $b in the variables area of the Formula tool:
// Add them!
$a + $b
Formula highlights:
No end of statement characters, such as a semicolon.
White space is ignored. Spaces, tabs, and line breaks should be used to improved readability, but have no effect on compilation.
The language is functional as opposed to procedural.
Variables start with an $ symbol and can be used to import data for use in a formula as well as improve readability by allowing the separation of a complex statement into intermediate statements.
Variables
Naming variables can be useful to increase readability of a complex or lengthy formula. Variables can be an alpha-numeric (plus the underscore character) name that is preceded by a $. In addition, they are able to be assigned only once, and must not start with a number. You can edit variable names by hitting the edit icon next to the variable.
Valid Variable Names | |
---|---|
CODE
|
CODE
|
CODE
|
CODE
|
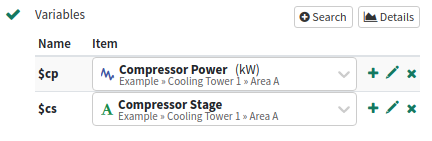
Functions
Formula has many built-in functions that seamlessly handle the difficulties of working with signal data. Interpolation, bad or invalid data handling, and maximum interpolation gap are all cases that are handled automatically by the base function set. In addition to functions that operate on signals, the list of built-in functions contains many useful functions for creating, modifying, and grouping conditions, capsules, markers, and other derived data products. The definitive list of functions can be found in Workbench.
Functions transform and, in rare cases such as signal generators, create data. Functions can be used in two styles: Excel and Fluent. Fluent and Excel styles may be used interchangeably in a calculation.
Scalar Style | Excel Style | Fluent Style |
---|---|---|
1m + 2m | ADD(1m, 2m) | 1m.add(2m) |
1m + 2m - 0.5m | SUBTRACT(ADD(1m, 2m), 0.5m) | 1m.add(2m).subtract(0.5m) |
(1m + 2m - 0.5m) * 2 | MULTIPLY(SUBTRACT(ADD(1m, 2m), 0.5m), 2) | (1m.add(2m).subtract.0.5).multiply(2) |
convertUnits($signal,"m") | $signal.convertUnits("m") |
The Excel style will be familiar to those who have spent time writing Excel formulas, as it uses the same nesting pattern where algorithmic steps are nested inside one another.
The Fluent style is intended to help with the readability problems that plague complex algorithms when expressed in the Excel style. In the Fluent style, the first parameter of a function is moved to the front of the function followed by a dot.
Fluent and Excel style mix-n-match | |
---|---|
SUBTRACT(1m.ADD(2m), 0.5m) | Equivalent to 1m + 2m - 0.5m. Produces 2.5m |
Lambdas
Lambdas are a valuable tool in functional programming that allows the user to define an inline function, and are used in Formula to specify behavior that is to happen within a function. For instance, the 'reduce' function makes use of a user-specified lambda to determine how to aggregate two elements of a group together; should it add them? subtract them? something else? By making use of a user-supplied lambda, many functions within formula gain significant flexibility as the full scope of what can be done within the function is limited only by the function set available within formula as a whole.
// $batches is a condition with a capsule for each batch. Here we want to remove any capsule that isn't at least 15 minutes in duration
$batches.filter($batch -> $batch.duration() > 15min)
Comments
Both single-line (// style) and multi-line (/* */) style comments are supported. Consider using comments to explain the thought process behind a particular segment of a formula, temporarily disable statements for debugging purposes, or notate authorship of a formula.
// This is a single-line comment
$a = 1m // These comments can even go at the end of a line
// They can even be used to disable statements from execution
// $b = 10m
/* Multi-line statements such
as this can span many lines */
$c = 100m /* They needn't span more than one line, though */
/* Just as with the single-line comments, these comments
can disable code from execution as well
$d = 1000m
*/
Return Value
A calculation must have one and only one return value. The 'return' keyword is optional, and, lacking a return keyword, the statement's value will be used. All of the below examples are valid calculations, and they all return 1 meter as their result:
1m
$a = 1m
return $a
$a = 1m
$a
$a = 1m $a
Literals
Numeric literals can be expressed in decimal, hexadecimal, or octal. In addition, numeric literals can be expressed with units. For a complete listing of available units see Seeq Units of Measure (UOM). Numeric literal examples:
10m // 10 meters
10 m // Also 10 meters
10.0m // Also 10 meters
-10.0m // Negative 10 meters
0.1m^2 // 1/10th of a square meter
.1m^2 // Also 1/10th of a square meter
0xAft // 10 feet expressed in hexadecimal
0xa ft // Also 10 feet
013 // Unitless 10 expressed in octal
-013ft // Negative 10 feet in octal
Time Literals
For time literals a ISO 8601 timestamp is used
YYYY-MM-DDThh:mm:ss.sssssssss±hh:mm
Where:
YYYY is the year as a 4 digit number
MM is the months as a 2 digit number
DD is the day as a 2 digit number
The T is used to separate the date and time elements.
hh is hour as a two digit number
mm are the minutes as a 2 digit number
ss are the seconds which can include fractional seconds
The ±hh:mm is the timezone offset from UTC. As a shorthand if the time is in UTC with no offset a Z can be used
e.g. '2016-01-01T19:51:32.490Z'.
String Literals
String literals are enclosed in quotes and may not span more than one line. A backslash character may be used to escape certain control characters, or, if many escaped characters are needed, it might be more convenient to use the escaped string form where the string is prefixed with an 'r' to denote all characters are literal and not part of an escaped character:
"A little pyramid: /\\" // Results in: A little pyramid /\
"I want a \" in my quote" // Results in: I want a " in my quote
'Single quotes may be used as well' // Results in: Single quotes may be used as well
'Using a " in here is fine, but you need to escape a \' for things to work'
"And a ' in here is okay too"
r"Since this string is preceded by an 'r' I needn't escape the backslash character: \"
Keyboard Shortcuts
When working in the formula editor, the following keyboard shortcuts are supported.
Keyboard Shortcut | Description |
---|---|
Ctrl+S (Windows & Mac) Cmd+S (Mac) | Executes the formula, like clicking on the "Execute" button. |
Ctrl+U (Windows & Mac) Cmd+U (Mac) | Shows dropdown which allows easy insertion of units. |
"." and Ctrl+Space | Shows autocompletion of functions. Please note that currently the autocompletion is simple, so it'll show all matching functions, even though they might not be valid for a specific variable type. |
F1 | Shows help for the function by the current cursor position. If an exact match isn't found a search in the help is performed instead. |